For some reason, using mipidsi
with textboxes from embedded-text
seems to produce a weird cut-off issue if the display has a pretty harsh offset. To preface, I'm unsure whether this issue is with mipidsi
or with embedded-text
, but it's probably best that the issue is tracked somewhere.
About the setup: I'm using an Adafruit Feather ESP32-S3 (TFT), with esp-hal. The TFT attached to the board is a 240x135 ST7789, with the SKU ADA4383
. From my testing with a filled box and a contrasting stroke colour, it seems to have similar offsets to the pico1
model, which is why that's used in my code below (along with mipidsi
0.5.0).
Text just appears to get cut-off at a seemingly arbitrary point on the display, no matter what. I have tried extending the text box, changing rotations, using different display models (in the code, not hardware), starting points, height modes, etc. None of them seem to allow text to be printed over this arbitrary cut-off point.
let di = SPIInterfaceNoCS::new(interface, dc);
let mut display = Builder::st7789_pico1(di)
.with_display_size(240, 135)
.with_orientation(Orientation::Landscape(true))
.init(Delay::get_delay_mut(), Some(rst))
.unwrap();
display.clear(Rgb565::BLACK).unwrap();
let text_style = MonoTextStyleBuilder::new()
.font(&FONT_9X15)
.text_color(Rgb565::WHITE)
.build();
let textbox_style = TextBoxStyleBuilder::new()
.alignment(HorizontalAlignment::Center)
.vertical_alignment(VerticalAlignment::Middle)
.build();
let bounds = Rectangle::new(Point::zero(), Size::new(235, 130));
let text_box = TextBox::with_textbox_style(text, bounds, text_style, textbox_style);
text_box.draw(display).unwrap();
The text below is supposed to say "1Player", but it doesn't show further than the P. It's not dead-centre as this was an attempt while I tried to use a wider textbox, but this issue persists no matter what.
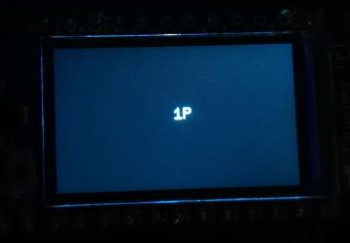
Solid colours still fill as expected, and below is a picture of a red fill (apologies for the bad photo, it is red). This box is using the exact same bounds
as the textbox, just with a solid red fill.
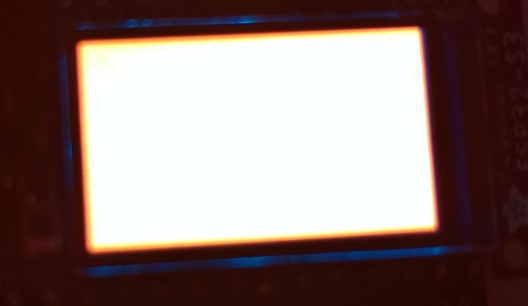