Concerns
Web MIDI is all kinds of asyncronous. Devices won't be available if you try to block request them immediately. It's possible that sending a MIDI device input immediately after opening it, if that input device requires system exclusive access, may not work. It'd perhaps be possible to buffer data until the promise for opening the device resolves...
Testing
C:\local\midir\examples\browser> wasm-pack build --target=no-modules --dev
...
C:\local\midir\examples\browser> "%ProgramFiles(x86)%\Google\Chrome\Application\chrome.exe" --allow-file-access-from-files --auto-open-devtools-for-tabs file:///C:\local\midir\examples\browser\index.html
Note that existing chrome instances may interfere with using command line flags - you may prefer to use the msjsdiag.debugger-for-chrome extension instead. Snippets from my local .vscode config:
.vscode\tasks.json
{ "label": "examples/browser: wasm-pack build --target=no-modules --dev", "options": { "cwd": "${workspaceFolder}/examples/browser" }, "windows": { "command": "wasm-pack build --target=no-modules --dev", }, },
{ "label": "examples/browser: wasm-pack build --target=no-modules --profiling", "options": { "cwd": "${workspaceFolder}/examples/browser" }, "windows": { "command": "wasm-pack build --target=no-modules --profiling", }, },
{ "label": "examples/browser: wasm-pack build --target=no-modules --release", "options": { "cwd": "${workspaceFolder}/examples/browser" }, "windows": { "command": "wasm-pack build --target=no-modules --release", }, },
.vscode\launch.json
// msjsdiag.debugger-for-chrome
// "runtimeArgs": See https://peter.sh/experiments/chromium-command-line-switches/
{
"name": "Chrome (--dev)", "type": "chrome", "request": "launch",
"preLaunchTask": "examples/browser: wasm-pack build --target=no-modules --dev",
"url": "${workspaceFolder}/examples/browser/index.html",
"runtimeArgs": ["--allow-file-access-from-files", "--auto-open-devtools-for-tabs"],
"internalConsoleOptions": "openOnSessionStart",
},
{
"name": "Chrome (--profiling)", "type": "chrome", "request": "launch",
"preLaunchTask": "examples/browser: wasm-pack build --target=no-modules --profiling",
"url": "${workspaceFolder}/examples/browser/index.html",
"runtimeArgs": ["--allow-file-access-from-files", "--auto-open-devtools-for-tabs"],
"internalConsoleOptions": "openOnSessionStart",
},
{
"name": "Chrome (--release)", "type": "chrome", "request": "launch",
"preLaunchTask": "examples/browser: wasm-pack build --target=no-modules --release",
"url": "${workspaceFolder}/examples/browser/index.html",
"runtimeArgs": ["--allow-file-access-from-files", "--auto-open-devtools-for-tabs"],
"internalConsoleOptions": "openOnSessionStart",
},
Screenshots
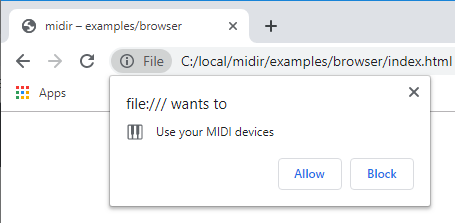
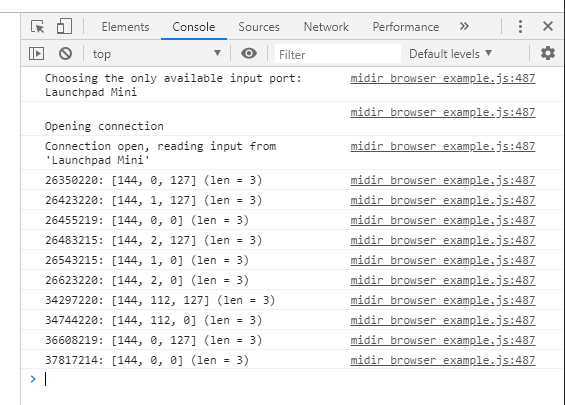